Learn PHP Fast! - A Beginner's Guide To Programming in PHP (How To Program Series)
Author: Paul Priddle
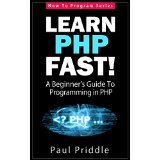
Book Series: Programming Languages
1. Introduction
If you are an aspiring programmer, or even someone with a fair amount of programming experience already, you must learn PHP. It is one of the most popular and powerful programming languages in use today. If you have hesitated because you thought PHP was too difficult to learn, then think again!
It is actually a fairly easy programming language to learn once you get used to the basic syntax and take a step-by-step approach to your learning process.
In fact, that is what this tutorial called Learn PHP Fast is designed to do. We have developed and organized this guide to get you up and running quickly with PHP. All you need to do is follow along, one section at a time, to learn all of the important fundamentals of PHP programming.
So what is PHP and why should you learn it?
What Is PHP
PHP (short for PHP: HyperText Pre-Processor) is one of the most popular scripting languages used on the web today. If you are looking to enhance your programming skills or wanting to develop your web design or programming career, then learning how to program using PHP code is definitely an invaluable skill to have.
PHP is a free and very popular Open Source scripting language that is used to create interactive and dynamic web pages very easily and quickly. Although HTML works very well for displaying fixed information, is it basically static and offers no user interaction. However, PHP can help bring a website to life and allow users to interact with a site. Some of the dynamic behaviors that PHP supports includes:
-
Generating dynamic page content
-
Offering website search facility
-
Providing user login and registration
-
Sending feedback via email
-
Collecting form data
-
Storing data inside a database
-
Displaying data stored in a database on a website
-
Receiving and sending cookies
-
Encrypting data
Surveys, forms, picture galleries, forums, ecommerce sites and much more can be created using PHP. You can also manage web applications, session tracking, databases and dynamic content.
PHP is a very flexible language and can co-exist with HTML content on a page as long as the page uses the .php extension. It also works with all of the popular databases, web browsers and web servers. PHP is a server-side scripting language, which means PHP scripts are executed on the web hosting server's server and not on a local computer's browser.
PHP is also a very powerful language. The extremely popular blogging platform WordPress was developed using PHP, and it also used for running Facebook, the largest social network in the world.
So as you can see, there are numerous reasons why learning PHP programming is an invaluable skill to have. Let's take a closer look at the topics we will covering in this tutorial.
In Learning PHP Fast, you will learn:
How to get started with PHP - learn what tools you need along with the basic syntax to get you off to a fast start.
PHP Variables - learn all about the "containers" that make all your PHP programming possible.
PHP Data Types - learn what the basic data types of PHP are and why they are important.
Constants - learn about values that never change and how you can use them to your advantage.
PHP Strings & Arrays - even more PHP options for you to learn about and use.
Operators - use these to perform mathematical functions and more.
Conditional Statements, Loops and Variable Scope - more tools for controlling the flow of your PHP programs.
Files and Forms - learn how powerful and flexible PHP can be when used with files and forms.
We cover all the basic building blocks of PHP in this tutorial. So what are you waiting for? Let's get started right now!
2. Getting Started With PHP
Installation and Set Up
Before you will be able to write PHP scripts and test them, there are a few things you will need to have. First of all, you will need a text editor or web design software. We recommend a simple text editor such as notepad for Windows users and TextEdit for Mac users. So go ahead and download a text editor or locate it on your computer. Notepad comes pre-installed on almost all Windows computers.
To view and test your PHP scripts, there are a few more things you will need to get set up. PHP is a server-side scripting language, which means you need to have a server to process the code. You cannot simply view it in your browser. In fact you need three things for you PHP programming project:
1. PHP programming language
2. Web server (i.e. Apache)
3. Database (i.e. MySQL)
There are two main ways to achieve this. The first way is to have a hosting account that provides PHP and MySQL support. Most hosting packages offer this. You can then simply upload your PHP files (with .php extension) to your hosting account using FTP software to view and test them.
The other option is to get PHP, a web server and MySQL database installed on your computer so that you can run and test your PHP scripts right from your own PC. When you are in the process of learning how to program in PHP, it is much easier to test on your own computer. Fortunately, there is an all-in-one solution called wampserver (mampserver for Macs) that will provide you will all of the three items that you need. They provide you with Apache Web Server, MySQL and PHP all in one installation.
To install Wampserver on a Windows-based computer, go to:
wampserver.com/en/download.php
For MAC users, go to:
mamp.info/en/index.html
Download the software and follow the installation instructions.
To test your installation type one of these two things into your web browser. It will let you know whether or not the web server is running:
http://127.0.0.1
http://localhost
PHP Syntax
Before we get into the various components of PHP programming, we want to cover basic PHP syntax in this getting started section.
Here is the basis structure that all PHP code and scripts follow:
PHP code
?>
You will be placing your PHP code within the opening tag of
PHP can be used as a script inside of an HTML document or an entire file can be written in PHP and named with a .php extension.
The following is a basic PHP file that includes a PHP script using the built-in PHP function of "echo" to output "Hello World" onto a web page. The PHP echo statement sends the text following the PHP statement to the web browser.
Example PHP File
Example PHP File
echo "Hello World";
?>
PHP Comments
PHP supports both single and multi-line comments. There are two ways to make single line comments and one way to make multi line comments.
// Here is one way to make a single line comment
# Here is another way to make a single line comment
/* A multiple line comment
spans more than one line
*/
PHP expression and statements are terminated by semicolons and PHP is case sensitive.
PHP is white space insensitive. This makes it possible to space out your PHP code and comments to make it more readable and understandable.
3. Variables
Variables are a very important aspect of a programming language. They allow you to store values in named containers to be used at a later date. So you can think of a variable as a container for storing such things as characters, strings of characters, numerical values or memory addresses.
You will frequently need to store data in your programs. When a program is executed, the data is stored by the computer processor in its memory cells until the data is used. Each memory cell will contain different data and has a number assigned to it. You might need to use the same data in your program multiple times. So each time you need to use the data in program, you're going to need the memory cell address because that is where the data is stored.
However, when you're working with lots and lots of data, trying to remember all of the cell numbers will be very difficult, if not impossible. So instead of trying to remember the cell numbers, cells where data is stored are assigned variables. For example, say you have the year 2014 stored inside the memory cell number 4938. Which one will be easier to remember, 4938 or "year?" It isn't necessary for you to know where 2014 specifically is stored. You just need to know what the name of the variable is ("year") to locate 2014.
Naming Rules for Variables
A variable name must start with $ (dollar sign): $year
The first character must be an underscore or a letter (not a number):
$_year or $year
The other characters in the variable name other the first character can have any amount of underscores, numbers (0-9) or letters (a-z, A-Z).
Variable names are case-sensitive. So, $Year and $year are two different variables.
Any variable not meeting any of the rules above is considered to be an invalid variable.
You should assign meaningful variable names so that you know what they stand for.
PHP does have predefined variables names. These begin with an underscore and then use all capital letters. For example, $_REQUEST. It is good practice to avoid using this format for your own variables to differentiate between your variables and the predefined ones.
How to Assign a Value to a Variable
There are two different ways that you can assigned values to variables: by reference or by value.
1. By Value
$variable_name = value;
The value can be any kind of data (for example, an integer, string, etc.).
For example, if you wanted to declare a value named year and assign the value of 2014 to it, here is the proper PHP syntax:
$year=”2014”;
?>
Assigning values to variables when declaring them is good practice.
2. By Reference
To assign a reference to a variable, use the following syntax:
$variable_name = &$existing_variable_name;
The variable $existing_variable_name refers to a variable that was previously declared and is used for referencing the variable called $variable_name.
For example, if you want to declare a variable called tomorrow and assign a reference of today to it, here is the PHP code you would write:
$today=“Tuesday”;
$tomorrow=&$today;
?>
Since the new variable references the existing variable, that means their values are identical. So when you change a variable's value it changes the other variable's value as well.
How to Display Variables
To display a variable, use one of the following:
print $variable_name;
or
echo $variable_name;
To display a variable called year, write the following:
$year=“2014”;
echo $year;
print $year;
?>
The output from lines 3 and 4 will both display 2014.
Types of Variables
In PHP there are two main types of variables: predefined variables and user-defined variables.
User-defined Variables
These variables are defined by the person writing the script. All of the examples above make use of user-defined variables.
Predefined Variables
PHP includes many predefined variables that are used for running scripts. This type of variable does play a very critical role in PHP programs. They provide useful information like information coming after a form has been submitted, server information, etc. Since this kind of variable is an associative array, we will cover predefined variables after going over arrays.
4. PHP Data Types
A data type in programming terms refers to a group of data having a common predefined set of characteristics. So for example, alphabetic characters are used to write your name and numbers are used to write your age. They are different types of information, so different data types are used to represent them.
There are 8 basic data types used by PHP. They are NULL, resource, object, array, string, float, integer and Boolean. These 8 basic types can be further categorized into 3 main types:
1. Single Valued (Scalar types)
String
Float
Integer
Boolean
2. Multi Valued (Compound Types)
Object
Array
3. Special Types
NULL
Resource
Boolean
Boolean data contains either a true or a false value.
For example:
$var= TRUE;
?>
Integer
An integer can be:
Any whole number not containing a fraction
An integer can be positive, negative or zero. Positive is the default.
Floating Point Number
Also referred to as real number, double or float. Fractions are not supported by PHP. Therefore, fractions are expressed in their equivalent floating point. So, 1 1/4 is expressed as 1.25.
A float can either be a positive number or a negative number. A positive number is the default.
String
Any sequence of characters is called a string. For example, "Hello World." It is any text that is placed inside quotes. Either double or single quotes can be used.
Example of a String:
$greeting = "Hello world!";
echo $greeting;
echo "
";
$greeting = 'Hello world!';
echo $greeting;
?>
Arrays
An array stores multiple values in one single variable. Values are mapped to keys. Array indexes or keys can be either strings or integers. Values can be of any type. We will be devoting an entire section to arrays later in this section, but here is a brief example:
$vegetables = array(
vegetable1 => "Peas",
vetetable2 => "Carrots"
);
$vegetables = [
vetetable1 => "Peas",
vegetable2 => "Carrots"
];
?>
Objects
The data type object not only stores data but also contains information on how the data should be processed. An object has to be explicitly declared, unlike other PHP data types.
First, the class of object must be declared. The class keyword is used for this. A class is a kind of structure that may contain methods and properties. Then the data type for the object class is defined. The type is then used in the instances of the class.
Example
{
function grades_calculation()
{
echo "Display grades";
}
}
$student1 = new student;
$student1->grades_calculation();
?>
Resource
A resource is a data type that references an external special resource outside of PHP. This could be an open database connection or file resource.
NULL
Null holds the value of nothing. It is not equivalent to zero, since zero is a value. It is also not equivalent to a blank space or empty string. Null refers to nothing. Any variable that hasn't been assigned a value yet, or that you've used the unset method on carries the NULL value. It can be useful when you are wanting to check whether a certain variable contains a value. You can compare the value against the NULL constant.
5. Constants
A constant is a name of a value. The value can be accessed later on in the script by using the name. If you have any values in the program you are writing that will not change anywhere in the script, it can be defined as a constant. An example of this would be the name of your website. Once that value has been defined, it cannot be undefined or changed later. The only place you change the constant is where it is defined.
How to Define a Constant
There are two different ways that you can declare a constant:
1. Use the define() function:
define(“CONSTANT_NAME”, value);
Example
define(“WEBSITE_NAME”,”My Awesome Website”);
?>
There is a third parameter that is optional. You can also add case insensitive after value if you don't want the constant name to be case sensitive:
define(“CONSTANT_NAME”, value, [case_insensitive]);
2. Use CONST keyword
const CONSTANT_NAME = value;
Example
const WEBSITE_NAME = “My Awesome Website”;
?>
Naming Rules for Constants
The naming rules for constants are the same rules that are used for variables except that you can't start a constant with a $. To be a valid constant, a name must adhere to the following rules:
1. The first character has to be an underscore or letter.
2. All other characters other than the first can have any number of underscores, numbers or letters (upper and lower case)
3. Constant names are case sensitive, unless the define() function is used and the third parameter of case_insensitive is set.
Data Types Supported By Constants
Only scalar data type values can be used with constants (string, float, integer and Boolean).
const IS_IT_YEAR_2012 = FALSE; // boolean value stored for constant IS_IT_YEAR_2012.
const DAYS_OF_WEEK = 7; // integer number value for constant DAYS_OF_WEEK.
const MONEY_REMAINING = 200.50; // float value for constant MONEY_REMAINING.
const WEBSITE_NAME = “My Awesome Website”; // string value for constant WEBSITE_NAME.
?>
Scope of Constants
Constants have global scope. Once you have defined a constant, it can be accessed anywhere in the PHP script.
If a different php script gets included after a constant has already been defined, that script can still access the constant.
Accessing a Constant's Value
There are two different ways that you can access a constant.
1. Use Print or Echo
Use print or echo in front of the constant to display it.
print CONSTANT_NAME;
or
echo CONSTANT_NAME;
echo CONSTANT_NAME;
?>
2. Use the constant() function
echo constant(“CONSTANT_NAME”);
echo constant(“WEBSITE_NAME”); // output: My Awesome Website
?>
Predefined Constants
There are many predefined constants that come with PHP. Any scripts being run by PHP can use these constants.
Magic Constants
Eight of the predefined constants are referred to as magic constants.
The constants are case sensitive and have two underscores at the beginning and end.
__NAMESPACE__
Name of current namespace
__METHOD__
Name of method containing the constant
__TRAIT__
Name of trait containing the constant
__CLASS__
Name of class containing the constant
__FUNCTION__
Name of function containing the constant
__DIR__
Script directory containing the constant
__FILE__
File name and full path where constant is defined. File name is shown if inside included file.
__LINE__
Script line number containing the constant
6. Strings
What Are PHP Strings
The PHP data type string is a collection of any amount of bytes or characters that are enclosed using string notation. The characters may be letters, spaces, variables and numbers.
How to Create Strings
In PHP, you can create strings in four different ways: Nowdoc syntax, Heredoc syntax, Double quote and Single quote.
Single Quote
With the single quote method, a single quotation mark is used for enclosing the characters.
The content that is inside the single quotes gets displayed as is. Variables within singe quotes do not get interpreted. The meanings of special characters inside single quotes are not expressed.
Example
$day_quantity=7;
echo ‘There are $day_quantity days. \n the first one is Monday.';
?>
Output:
There are $day_quantity days. \n the first one is Monday.
In the above output, the value of the variable isn't printed and the output isn't effected by the new line \n special character.
Double Quote
For expressing a string, the double quote is used the most.
Double quotation marks (" ") enclose the characters.
Special characters and variables are interpreted by PHP interpreter when they are inside double quotes. Compare the following example with the one above:
Example with Double Quotes
$day_quantity=7;
echo "There are $day_quantity days. \n the first one is Monday.";
?>
Output:
There are 7 days.
The first one is Monday.
The string must be enclosed by either two single quotes or two double quotes. If you have one single quote and one double quote, it will produce a syntax error.
Displaying a double quote or single quote within a string.
Here is how you can display an apostrophe (single quote) within a string with double quotes:
echo “This is Bill’s site.”; // Output: This is Bill’s site.
?>
Here is how to display double quotes within a string enclosed by single quotes:
echo ‘Bill said “That is my cat”.’; // Output: Bill said " That is my cat".
?>
Here is how to display double quote within a string enclosed by double quotes:
echo “Bill said \”That is my cat\”.”; // Output: Bill said "That is my cat".
?>
This same rule can be applied with single quoted strings as well:
echo ‘Bill said \’That is my cat\’.’; // Output: Bill said ‘That is my cat’.
?>
Heredoc Syntax
Using Here-document (or heredoc) syntax is the third way to create strings. Heredoc is the best choice when you need to display a large amount of text.
It works in a similar way to double quotes except that escaping quotes are not required.
Heredoc Syntax Rules
Heredoc syntax starts with 3 less than signs (<<<). This is followed by the user-defined name. Any combination of underscores, numbers and letters can be used in the name. However, the first character needs to be an underscore or letter.
The string starts on the next line.
Following the string, the exact same name defined after <<< in first line must be placed on the next line by itself. It is option to use a semicolon following the name.
Example:
echo <<
This is the first line. \nThis is the second line.;
EOT;
?>
Output:
This is the first line.
This is the second line.
EOT and EOD are the most commonly used Heredoc names. The heredoc name should be coded in all caps.
Nowdoc Syntax
Nowdoc syntax works in a similar way that single quotes do, just like heredoc syntax works in a similar way to double quotes.
The variable inside a nowdoc is not interpreted, just like with singe quotes.
Nowdoc Syntax Rules
All the Heredoc syntax rules are supported by Nowdoc except that single quotes must enclose the starting name.
Example:
echo <<<‘EOT’
This is the first line. \nThis is the second line.;
EOD;
?>
Output:
This is the first line. \nThis is the second line.
7. Arrays
In PHP, an array is an ordered map where values are associated to keys.
If your program needs to have a day of the week in it (for example, Monday), you can declare a variable name and then assign the day of the week in it. For example:
$day=“Monday”;
?>
Monday is a string data and surrounded by quotes.
If you need to add all of the names of the week, there are two different ways for you to do this.
Method 1: Declare 7 different variable names and assigns days of the week names for those variables:
$day=“Monday”;
$day2=“Tuesday”;
$day3=“Wednesday”;
$day4=“Thursday”;
$day5=“Friday”;
$day6=“Saturday”;
$day7=“Sunday”;
?>
However, this is a time consuming and boring way to go about this.
Method 2: Using method 2 offers a better solution:
$day=array(“Monday”, “Tuesday”, “Wednesday” , “Thursday” , “Friday” , “Saturday” , “Sunday”);
?>
Arrays Defined
An array is a data type where multiple values are represented. In our example above, the array represents 7 day of the week names. The array name is "day." The data type’s integer, float, string and Boolean all represent just one value. An array, on the other hand, represents multiple values.
Terms Commonly Used With Arrays
Element: the items contained in an array. There can be one or more elements contained in an array.
Value: every element has one value.
Index or Key: an array's index or key is a unique string or number associated with each of the element's values.
Length: An array's length is how many elements are contained in the array.
Creating an Array
You can create an array in two different ways:
1. Use array() function.
$array_name = array(value1, value2, value3, ...);
Example
When an array is created as above, automatic index numbers begin with 0 and increase by 1 for each subsequent value. The first value "Monday" has a 0 index number, Tuesday has an index number of 1 and so forth.
Creating Index Numbers Manually
When creating arrays, there is a variation for the array() function. With this method, you can mention the key/index number when you declare the values.
array_name = array(
index1 => value1,
index2 => value2);
Example
$day = array(
0 => “Monday”,
1 => “Tuesday”,
2 => “Wednesday”
);?>
2. You can also use the [] identifier for creating an array.
$array_name[] = value;
$day[]=“Monday”;
$day[]=“Tuesday”;
$day[]=“Wednesday”;
?>
In the example above, Monday has a 0 index, Tuesday has an index of 1 and Wednesday has an index of 2. The key/index can be assigned within []:
$array_name[key] = value;
Example
$day[0]=“Monday”;
$day[1]=“Tuesday”;
$day[2]=“Wednesday”;
?>
You cannot assign array keys arbitrarily. The missing elements won't be filled up by PHP. In the example below, PHP will leave $animal(3). When you add another element, that element won't be inserted into $animal(3) by PHP. The new element's key for giraffe would instead be the next number following the existing key. In this case, it would be 5;
$animal[0]=‘Dog’;
$animal[1]=‘Cat’;
$animal[2]=‘Fish’;
$animal[4]=‘Horse’;
$animal[]=‘Giraffe’;
?>
How to Change an Array's Values
To change an element's existing value, the new value needs to be specified in the array. The element's key is mentioned in [] brackets.
$array_name[existing_key] = “new_value”;
Example
$day = array(
0 => “Monday”,
1 => “Tuesday”,
2 => “Wednesday”
);
$day[2] = “Sunday”;
?>
Deleting an Array
To delete either the entire array or an array element, you can use the unset() function.
$animal = array(“Dog”, “Cat”, “Fish”, “Horse”);
unset($animal[2]); // Array elements are now Dog, Cat, NULL, Horse
unset($animal); // Array elements are now all NULL
?>
Array Types
There are two different kinds of array types:
1. Associative Array
2. Indexed Array
Indexed Array
Indexed arrays are arrays with a numeric index/key. It's the most common array type. All the arrays we have used up to this point in our examples have all been indexed arrays.
Associative Array
Associative arrays use a string instead of a number for the index/key.
Multidimensional Arrays
When an array's key represents another array, it's a multidimensional array. All of the example of array above had one value for each key.
Example
$brothers = array(
array(“Joe”, 25, “CA”),
array(“Jeff”, 20, “FL”)
);
?>
How to Print Array Elements
To print "Monday" from our "day" array above, write the following:
echo $array_name[key];
echo $day[0];
?>
Output:
Monday
8. Operators
In programming language like PHP, operators are symbols that help with performing different kinds of operations such as arithmetic, logic and relations.
Operators
Operators are symbols like , -, /, *
Based on a predefined rule, operators can take an expression or value on the right or left or both sides to produce a result.
For example, the operator adds the values on each side:
2 + 3 = 5
Operands
Operands refer to data that you can manipulate.
Operands can be a literal (ex. 2), strings (ex. Tuesday), variables, constants or expressions.
Expressions
An expression is anything that yields a value:
$var = 5;
?>
In the example above, 5 is a value itself, which also makes it an expression. 5 is assigned to $var, so its value is 5. So $var is an expression also.
An expression can consist of operators and operands:
$var = 3+5;
?>
Types of Operators
There are three categories of operators, depending on how many values or expressions they take.
1. Unary Operators
This type of operator takes on just one value. They include: logical not operator (!), decrement/increment operators, error control operators, bitwise not operator (~) and arithmetic negation operator (-).
2. Binary Operators
This type of operator takes on two values. They include: array operators, string operators, logical operators, comparison operators, assignment operators and arithmetic operators.
3. Ternary Operator
Takes three values
Arithmetic Operators
Mathematical calculations are performed with arithmetic operators
Arithmetic operators include: addition ( ), subtraction (-), division (/), multiplication (*), and modulus (%).
In addition, PHP comes with many predefined mathematical functions that can perform different mathematical operations.
Arithmetic Operators Example
$a = 3;
$b = 2;
echo -$a; // -3
echo $a + $b; // 5
echo $a - $b; // 1
echo $a * $b; // 6
echo $a / $b; // 1.5
?>
Assignment Operators
The value of an expression is set by an assignment operator.
The main assignment operator is =
Example of an Assignment Operator:
$today = “Tuesday”;
?>
The assigning operator is =. It assigns the value of Wednesday to the variable $today.
To assigns values in array keys, you use the operator =>
Example
$day = array(
0 => “Monday”,
1 => “Tuesday”,
2 => “Wednesday”
);
?>
Combined Operators
Assignment operators can be combined with arithmetic, array union and string operators in PHP. Using combined operators allows you to perform calculations on variables. Then the result can be reassigned to the variable.
Example:
$a = 4;
$a += 1; // result: 5
?>
Comparison Operators
Two expressions or values are compared. Either true or false is returned. Here are some examples of comparison operators:
Equal $a == $b;
Identical $a === $b;
Not equal < >
Greater than >
Less Than <
Logical Operators
Help with making decisions whether something is true or false depending on what the expression or values are.
Frequently logical operators are used with statements and other control structures. Here are some examples of logical operators:
And $a and $b
If $a and $b are both true, true is returned. If not, false is.
Or $a or $b
True returned if either value is true. If not, false is.
Not !$a;
True returned if $a is not true. If not, false is.
String Operators
Concatenation operator (.) - joins two strings together. $a . $b;
Concatenating assignment operator (.=)- appends arguments on right side to left side:
$a.= $b; is equivalent to $a= $a.$b;
Example
$a= “Dog “;
$b = “Cat”;
$c = $a . $b; // Output: Dog Cat
$a .=$b; // Output: Dog Cat
?>
Array Operators
Union $a + $b
array $a is joined with array $b
Equality $a == $b
True is returned if arrays $a and $b have same value/key pairs
Identity $a === $b
True is returned if array have same value/key pairs in same order and data type is identical
Inequality $a <> $b
True is returned if arrays are not the same.
Non-identity $a !== $b
True is returned if arrays are not identical.
9. Conditional Statements
Conditional statements are critical for dynamic web applications. In PHP, a program's flow can be altered by conditional statements.
Control Structures
As we go about our lives, the actions we take can change depending on what the circumstance is. For example, if it is snowing, you might wear a coat and gloves instead of going out in a t-shirt and shorts.
When it comes to programming languages like PHP, control structures determine the flow of execution/action of the program. A control structure is a block of statements. PHP has several different control structures. We will be discussing these in this section.
Conditional Statements
In PHP, the flow of the program changes depending on what the condition is. The constructs controlling the program to make a decision is referred to as conditional statements. In programming, when a condition is true, then one or more statements get executed. When the condition is false, a different statement gets executed instead.
if statement
Syntax: if (expression)
If the expression is found to be true, then the statement following the parenthesis gets executed. Otherwise, it is not executed.
Example:
$snowing = “yes”;
if($snowing == “yes”){
echo “Wear gloves.”;}
?>
Output:
Wear gloves.
if statement alternative syntax:
if(expression):
statement;
endif;
$snowing = “yes”;
if($snowing == “yes”):
echo “Wear gloves.”;
endif;
?>
Output:
Wear gloves.
else statement
In real life, sometimes there is an alternative option that can be taken when a condition isn't met. For example, when it snows, you put on gloves; otherwise, you wear shorts. In PHP, you use the else statement for an alternate option. When the expression isn't true in the statement, then the else statement is executed by the program.
Syntax for else statement:
if (expression)
statement;
else
statement;
Example:
$snowing = “no”;
if($snowing == “yes”){
echo “Wear gloves.”;
} else {
echo “Wear shorts.”;}
?>
Output:
Wear shorts.
In an else statement has more than one statement to execute, then curly braces are used to enclose the statement.
Example:
$snowing = “no”;
if($snowing == “yes”){
echo “Wear gloves. “;
echo “
“;echo “Stay home.”;
}
else{
echo “Wear shorts. “;
echo “
“;echo “Go on a picnic.”;
}
?>
Output:
Wear shorts.
Go on a picnic.
Note: when the if statement is true, the else statement does not get executed.
else-statement alternative syntax:
if (expression):
statement;
else:
statement;
endif;
Example:
$snowing = “yes”;
if($snowing == “yes”):
echo “Wear gloves. “;
echo “
“;echo “Stay home.”;
else:
echo “Wear shorts. “;
echo “
“;echo “Go on a picnic.”;
endif;
?>
Output:
Wear gloves
Stay home.
elseif statement
In PHP, the elseif statement is used when there is more than one option.
Syntax for elseif statement:
if (expression)
statement;
elseif (expression)
statement;
else
statement;
When the if... statement is false, then the else statement is executed instead of the if statement.
Example:
$day = “Saturday”;
if($day == “Saturday”)
echo “Wear black pants.”;
elseif($day == “Sunday”)
echo “Wear red pants.”;
elseif($day == “Monday”)
echo “Wear blue pants.”;
else
echo “Wear white pants.”;
?>
Output:
Wear black pants.
Sometimes more than one statement may need to be executed. In those cases, curly braces enclose the statements.
Example:
$day = “Monday”;
if($day == “Saturday”){
echo “Wear black pants.”;
echo “
“;echo “Go out with friends.”;
}elseif($day == “Sunday”){
echo “Wear red pants.”;
echo “
“;echo “Go to church.”;
}elseif($day == “Monday”){
echo “Wear blue pants.”;
echo “
“;echo “Go to work.”;
}else{
echo “Wear white pants.”;
echo “
“;echo “Go to school.”;
}
?>
Output:
Wear blue pants.
Go to work.
elseif statement alternative syntax:
if (expression):
statement;
elseif (expression):
statement;
else:
statement;
endif;
Example:
$day = “Saturday”;
if($day == “Saturday”):
echo “Wear black pants.”;
echo “
“;echo “Go to party.”;
elseif($day == “Sunday”):
echo “Wear red pants.”;
echo “
“;echo “Go to church.”;
else if($day == “Monday”):
echo “Wear blue pants.”;
echo “
“;echo “Go to work.”;
else:
echo “Wear white pants.”;
echo “
“;echo “Go to school.”;
endif;
?>
Output:
Wear black pants.
Go to party.
Switch Statement
A switch statement can be used when there are many options to choose from.
Syntax of a switch statement:
switch (expression) {
case label:
statement(s);
break;
case label:
statement(s);
break;
....
default:
statement(s);
}
The label must be a string or number and the labels all end with a colon.
After a case label, there can be one or multiple statements.
Break is used for terminating out of the switch. The program continues to execute until a break statement is reached.
How a switch statement works:
The expression's value following the switch gets matched to the labels.
Once a match has been found, the case's subsequent statements are executed until the break statement is reached.
If no match is found with any of the labels, the optional default statement is executed.
Example
$day = “Monday”;
switch ($day) {
case “Saturday”:
echo “Wear black shirt.”;
break;
case “Sunday”:
echo “Wear red shirt.”;
break;
case “Monday”:
echo “Wear blue shirt.”;
break;
default:
echo “Wear white shirt.”;
}
?>
Output:
Wear blue shirt.
10. Loops
A loop allows a statement(s) to be executed numerous times until a condition is fulfilled.
What if you need to print the phrase "I want to learn php" four times? You could use the following PHP code:
echo “I want to learn php”;
echo “I want to learn php”;
echo “I want to learn php”;
echo “I want to learn php”;
?>
However, what if you needed the above sentence printed 100 times? When you want to have to write it out in your program 100 times? In programming, the loop feature can be used for performing repetitive tasks.
PHP has several different loops that can be used. We will cover these in this section.
1. While Loop
In PHP, this is the simplest kind of loop.
Syntax for while loop:
while (expression)
statement;
How a while loop works:
When the while keyword is reached by the php interpreter, the expression's value inside the parentheses is evaluated.
The next statement is executed if the expression is true. The interpreter goes back to the while keyword and checks the expression again. The loop continues until the expression's value following the keyword is false.
If the loop will be executing more than one statement, then brackets can be used to enclose the statements:
while (expression){
statement1;
statement2;
....
}
Example:
$i =1;
while($i<3){
echo “I want to learn php.”;
echo “
“;$i++;
}
?>
Output:
I want to learn php.
I want to learn php.
2. do-while loop
This type of loop is slightly different than the while loop.
With the while loop, the loop doesn't run the first time it is checked if the expression is false. With the do-while loop, the loop runs one time at least. So the first time it is checked, it is executed whether the expression is true or false.
Syntax of do-while loop:
do
statement
while(expression);
If the loop needs to execute more than one statement, brackets can be used to enclose the statements:
do{
statement1;
statement2
...
}while(expression);
Do-while loop example:
$i =1;
do{
echo “I want to learn php.”;
echo “
“;$i++;
}while($i<3)
?>
Output:
I want to learn php.
3. for loop
In PHP, the most complex loop is the for loop.
Syntax of the for loop:
for(expression1; expression2; expression3)
statement;
Expression 1 is the initialization expression. It sets the counter's value. The counter's function is to count the number of times that the loop iterates. This expression is only executed one time.
Expression 2 is the condition expression. Prior to each iteration, this expression tests whether the loop needs to stop or continue.
Expression 3 is the modification expression. Following each iteration of the loop, this expression determines how to alter the loop counter. It can be decremented or incremented.
How the for loop works:
1. When the for keyword is reached by the php interpreter, expression1 is executed and the counter's value is set.
2. Expression2 is then checked by the interpreter to determine whether or not the loop should continue. When expression2 is true, the loop will continue. If it is false, the loop is stopped.
3. Expression3 is then executed. The counter is either decremented or incremented.
4. Then the interpreter returns to step 2.
If the loop has more than one statement that needs to be executed, brackets can be used to enclose the statements:
for(expression1; expression2; expression3){
statement1;
statement2;
....
}
Example:
for($i=1; $i<3; $i++ ){
echo “I want to learn php.”;
echo “
“;}
?>
Output:
I want to learn php.
I want to learn php.
4. foreach loop
This type of loop was designed to specially access all of an array's elements in the easiest and fastest way. The foreach loop only works in objects and in arrays.
syntax of the foreach loop:
foreach($array as $value)
statement;
Use the following syntax when the key for all of the array elements need to be accessed:
foreach($array as $key => $value)
statement;
How the foreach loop works:
1. When the foreach loop is reached by the php interpret, the array's first element is taken and placed into the $value.
2. The statement immediately after the closing parentheses is then executed.
3. The interpreter returns to the first step.
The process continues until all of the array's elements have been used.
If the loop has more than one statement that needs to be executed, brackets can be used to enclose the statements:
foreach($array as $value){
statement1;
statement2;
....
}
foreach loop example:
$day=array("Monday", "Tuesday");
foreach($day as $value){
echo $value;
echo "
";}
?>
Output:
Monday
Tuesday
Break Statement
This is used for ending the following control structure's current execution: switch, do-while, while, foreach and for.
Example:
$day=array("Monday", "Tuesday", “Wednesday”, “Thursday”, “Friday”, “Saturday”, “Sunday”);
foreach($day as $value){
echo $value;
echo "
";if($value == “Thursday”)
break;
}
?>
Output:
Monday
Tuesday
Wednesday
Thursday
Continue Statement
This is used for ending the following control structure's current execution: switch, do-while, while, foreach and for.
It doesn't execute the current loop's remaining statements. Instead, it checks the loop's condition to start on the next iteration.
Continue statement Example
$day=array("January", "February", "March", "April", "Wednesday", "May", "June");
foreach($day as $value){
if($value == "Wednesday")
continue;
echo $value;
echo "
";}
?>
Output:
January
February
March
April
May
June
11. Working with Files and Reusing Your PHP Code
The PHP scripts we have been working with in this tutorial have been fairly short and simple. However, out in the real world, it will be a different story.
When you are working with large websites, you will discover that you end up using some of the same code over and over again in various scripts. As an example, websites tend to use the same header, navigation menu and footer on each page. It can get tedious and time-consuming to have to copy and paste the same code for the header on each page of the website.
Fortunately, in PHP, you can use a separate script to write the header code to include on all the separate pages of the website. There is a feature of PHP called server-side includes. This allows you to incorporate one script's code into a different script.
PHP has four different functions that can be used for incorporating external files: require_once(), require(), include_once() and include().
1. include()
When you use the include() function into a current script, it's the equivalent of pasting the included file's content in.
Syntax for include():
include(‘./path/to/the/filename’); (parentheses are optional)
Example:
The following is contained in webpage.php
echo "This content is from webpage.php.";
include "./hello.php";
?>
The following is contained in hello.php:
echo "
";echo "This content is from hello.php.";
?>
Output:
This content is from webpage.php.
This content is from hello.php.
2. require():
The require() function works the same way that include() does. The only difference between the two is if the the specified file does not exist. For example, if it was written as hellow.php instead of hello.php. With the include() function, a warning would be shown and the remainder of the page would be executed. With the require() function, a warning is shown and it stops executing the page.
If the main script cannot run unless the specified script is used, then required() should be used. Otherwise, it is usually best to use include().
3. include_once()
This works in a similar way to include(). The difference is that it first checks to see whether or not the file has been included already. Sometimes the same file might be called more than one time accidentally. If the file already exists, then include_once() won't include it again.
include_once() syntax:
include_once(‘./path/to/the/filename’);
The same rules used for include() are used with include_once().
4. require_once()
This works in a similar way to require(). It first checks to see whether or not the file has been included already. If so, it doesn't include it again.
require_once() syntax:
require_once(‘./path/to/the/filename’);
The same rules used for require() are used for require_once().
Advantages to Reusing Your PHP Code
There are several advantages to setting up a separate file for PHP code that you are going to be using in multiple places in your project. Here are the three biggest ones
Reliability: You can test your code and make sure it is bug free before using it multiple times. If you instead write new code, it will need to be tested each time and is more likely to have bugs in it.
Cost: When you reuse code, there will be less code overall. It will take less time to code, test, maintain, modify and update. Overall, this helps to reduce programming and maintenance costs tremendously.
Time: reusing code will help to reduce development time significantly.
12. Functions
Why PHP Functions Are Useful
Sometimes you will have a project that needs to perform the same task numerous times. It can get very time-consuming and tedious to write the same PHP code each time it is needed by the program. To make this an easier process, the function programming feature was developed. The function allows you to define a set of statements for performing one task. Then you can call the function by name anytime you need to use it.
One standard function defines a single task.
The Function Defined
A function is a named block of code:
That's defined to perform one task.
Can be called in from a different part of the program.
Might or might not return a value.
Advantages to Using Functions
Less code: You only have to write a function once.
Less execution time: You can call the function many times on a page. However, it only has to be compiled one time.
Fewer bugs: Since you only need to write the function one time, it will reduce the number of bugs and time needed for testing.
Improved readability: Since the function is a separate piece of code for performing a specific task, readability is improved for anyone wanting to understand exactly how the function is written and executed.
How to Define a Function
Syntax for a Function:
function function_name(parameter_1, parameter_2, ..., parameter_n){
// statements
}
Example
function welcome(){
echo “Hello, welcome to our Website.”;
}
?>
The function above performs a single task. When the function is called, the message "Hello, welcome to our Website" is displayed.
Second Example
function combine_names($f_name, $l_name){
echo $f_name . “ “ . $l_name;
}
?>
This function includes two parameters. The function combines the first and last name and prints them.
Rules For Naming Function
You want your function names to be meaningful so that it is clear what it does.
Each function name must be unique.
Valid function names start with an underscore or letter followed by underscores numbers and/or letters.
A function name is case sensitive.
How to Call a Function
It is easy to call a function. You just write the function's name followed by a set of parentheses.
function_name();
Once the function has been called, the statements that are inside the function get executed.
Once the execution of the function is complete, the program control goes back to the function.
Function Arguments
When a function that you call accepts inputs, the values are separated by commas. The values are referred to as arguments.
concat_names(“Bill”, “Watson”);
?>
Function Parameters
The inputs within the function definition parentheses are the parameters.
function concat_names($f_name, $l_name){
echo $f_name . “ “ . $l_name;
}
concat_names(“Bill”, “Watson”);
?>
In the example above, $f_name and $l_name inside the parentheses are the parameters.
When a function gets called that has arguments, the arguments are passed to their corresponding parameters.
So in this case "Bill" is passed to $f_name and "Watson" is passed to $l_name.
If there are more argument than parameters, then the extra argument are just ignored.
If there are fewer arguments than parameters, a warning will be issued that arguments are missing.
How to Pass Arguments
Arguments can be passed by reference or by value.
13. PHP and Variable Scope
Scope refers to the portion of a PHP script where a variable can be accessed after it's declared. The declaration's location determines the variable's scope.
A scope may be divided into two different parts, depending on whether the script has a function or not.
1. Function scope/local scope
Function or local scope starts at the function's declaration to the end of the function's closing brace.
2. Global scope
The function's remaining parts, other than local scope, are included in global scope.
The scope of a variable changes, depending on where the declaration is located. There are four different types of variables:
Function parameters, static variables, global variables and local variables.
Local Variables
When variables are declared within a function, they are local to the function.
The scope is from the place where it's declared to the end of the function's closing brace. When the function is terminated the variable dies. The variable cannot be accessed outside of the function.
Local Variable Example:
function website_name(){
$name = “My Website”;
}
?>
In the above example, the variable $name was declared and assigned within the function. So $name is a local variable of this function.
Global Variables
A variable that is declared outside of a function in a script is a global variable.
The scope of a global variable exists from the place that it's declared all the way to the ending of the script, except for any functions that are declared inside that area.
When the script is terminated, the variable dies. Global variables aren't accessible within functions.
Here is an example:
$name = “I Love PHP”;
function website_name(){
echo $name; // an error will be generated
}
website_name();
?>
When we attempt to print the variable within the function, an error is generated. This is because the variable $name is a global variable that was declared outside of the function.
Static Variables
Once a function is terminated, the local variables within the function are destroyed. When the keyword static is placed before a local variable, the value of the variable can be retained. The value is remembered from one call of the function to another one in the script. This type of variable is referred to as a static variable.
The scope starts at the place where the variable is declared and ends at the ending of the function's closing brace. When the script is terminated, the variable dies.
The static variable's value is only initialized one time when the function is first called.
Example:
static $val=1;
Function Parameters
Whenever a function gets called that has arguments, the values are passed to the parameters. Therefore, variables are declared within the parameters.
The scope starts at the place where it's declared and ends at the ending of the function's closing brace.
When the script is terminated, the variable dies.
14. Forms
Forms are an essential component in web development. They are used as a way for users and a server to communicate with each other. A form can be used to get a user's information sent to the server and allow the server to respond to the input provided by the user. Forms can be used to register at a website, to send feedback to login, to place an order and many other things.
In this section of the tutorial, we will cover how PHP handles forms.
How Forms Work With PHP
Usually forms are coded in HTML. The user fills in his information and then submits the form. This information gets sent to a designated PHP page. There the user inputs can be retrieved by the PHP. Here is the entire process broken down into steps:
1. Build the HTML form
2. Choose a form method for sending form inputs
3. Retrieve form inputs
4. Validate form inputs
5. Sanitize form inputs
Build Form
Here is a snippet of code from a basic form:
The code snippet for the form above has two main elements, a submit button and a text box.
How to Choose a Form Method for Sending Form Data
Two different methods are available for specifying how form data gets passed to the PHP script located on the server:
POST method and GET method.
The post method is used in the example above.
1. GET Method
This is the default method for a form. If a method isn't specified in the form, the information will be sent using the GET method.
When the GET method is used and the form is submitted, all of the form parameters (the values and names of the form elements) get added to the URL on the end.
For example, if you added the name Jack and then clicked submit on our example form above, this is how the URL would look:
/form-processing.php?name=Jack&send=Submit
These URLs do have size limitations, so you cannot use the GET method to pass on an unlimited amount of data via a form.
2. POST Method
When the POST method is used, after the form is submitted, form parameters get passed internally and the URL is not touched.
Any amount of data can be passed using the POST method.
When the GET Method Should Be Used
You can use the GET method for retrieving information from a server.
For example:
Logging into a member's area
Search forms
When the POST Method Should Be Used
Use the POST method anytime the processing script might change the database.
For example:
Updating user information
Placing orders in the shopping cart
Sending feedback
Registering at a website.
How to Retrieve Form Inputs
The retrieving process takes place in the PHP script specified in the action attribute of the form. In the form above, the processing script is at:
form-processing.php.
The submitted form data is stored inside Superglobal arrays. These are associative arrays and can used with any scope for retrieving form data. These Superglobal array are used for processing form data:
$_GET:
All data sent via the GET method is stored inside the $_GET associative array.
There are the same amount of elements in $_GET as elements in the form.
Each of the array element indexes is the name of one of the forms fields. The value is the same value that was entered into the field on the form.
Here is our form again:
This is the form processing script at form-processing.php:
$first_name = $_GET[‘first_name’];
$send = $_GET[‘send’];
echo $first_name;
echo “
“;
echo $send;
?>
Output:
Jack
send
$_POST:
All the data that gets sent via the POST method gets stored inside the $_POST associative array.
There is the same amount of elements in $_POST as number of form elements.
Each of the array element's indexes is the name from one of the form fields and the value is the value that was entered into the form field.
This is the form processing script at form-processing.php:
$first_name = $_POST[‘first_name’];
$send = $_POST[‘send’];
echo $first_name;
echo “
“;
echo $send;
?>
Output:
Jack
send
In both methods, the form data can be retrieved using its name index.
$_REQUEST:
All data sent by POST or GET method gets stored inside the associative array $_REQUEST.
There is the same amount of elements in $_REQUEST as number of form elements.
Each of the array element indexes are the name of a form field. The value is the same value entered into the field on the form.
This is the form processing script at form-processing.php
$first_name = $_REQUEST[‘first_name’];
$send = $_ REQUEST [‘send’];
echo $first_name;
echo “
“;
echo $send;
?>
How to Validate Form Data
It is necessary to validate form data, because a user might enter garbage or invalid data into a form. You have to ensure that the data is formatted properly.
There are various methods available for validating for data. Below, we will discuss a few of them.
Here is the form we will be using:
Name and username are both required in the form above. They must be 5 characters long at least. Now we will write a processing script in PHP at form-processing.php so that we can validate these four conditions:
The user's name was added
The user's username was added
The username is 5 characters long at least
The submit form was clicked
// Check to see if form button was submitted
if(isset($_POST[‘send’])){
if(!empty($_POST[‘name’])){ // Check if name field is empty
echo $first_name = $_POST[‘first_name’];
}else{
echo “Missing Name.”;
echo “
“;
}
if(!empty ($_POST[‘username’])){ // Check if username field is empty
if(strlen($_POST[‘username’]) < 5 ){ // Check if username is 5 characters at least.
echo “Your Username must be a minimum of 5 characters.”;
echo “
“;
}else{
echo $name = $_POST[‘username’];
}
}else{
echo “Missing Username.”;
echo “
“;
}
}else{
echo “You are not authorized to access this page.”;
}
?>
Checking Whether the Submit Button Was Clicked
Here is the code for checking whether the submit button was clicked or not:
if(isset($_POST[‘send’]))
The built-in function isset() takes a variable as a parameter and then checks whether it is set. We use the submit button as our variable and check to see whether or not the submit button was clicked.
If not, the user will get the message "You are not authorized to access this page."
Check Whether User's Name Has Been Entered or Not
PHP has a function called empty() that checks whether or not a variable is empty. We can use that to see whether or not the first name field in our form is empty. If the name field is filled out, the name is printed on the next line. If not, the function returns false. The else statement gets executed. "Missing name" gets printed.
Check Whether Username Has Been Entered or Not
The empty() function is used for this as well in the same way as above.
Check Whether Or Not the User Name Is 5 Characters Long At Least
The PHP function strlen() can tell if this is actioned correctly.